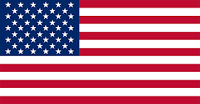

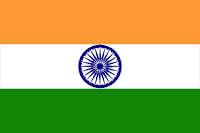
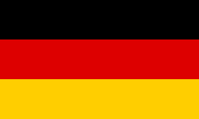
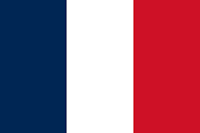

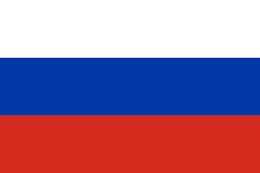
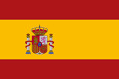



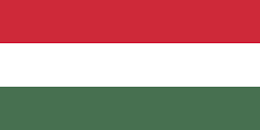
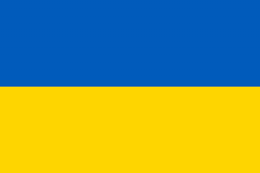
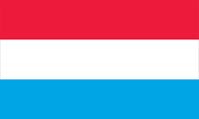

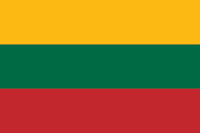

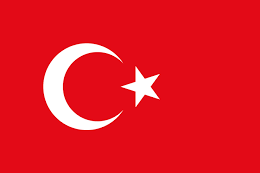


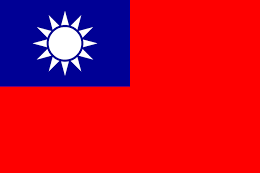
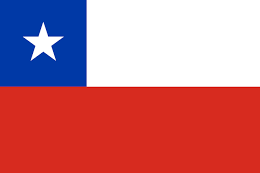

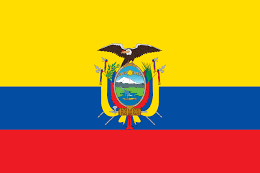
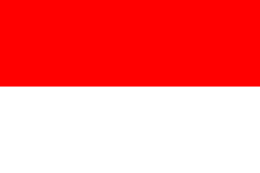
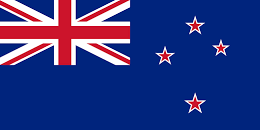
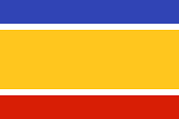
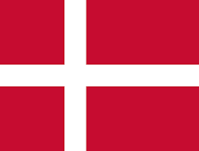
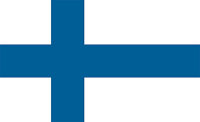


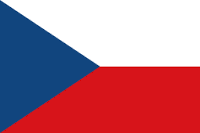
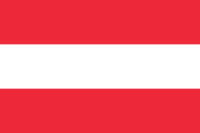
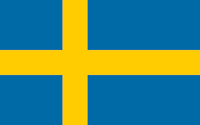
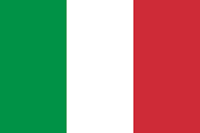
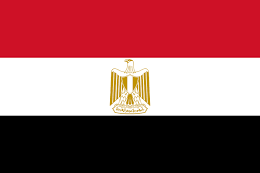

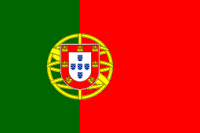
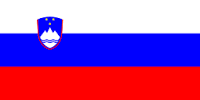

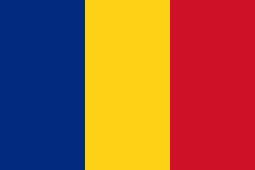
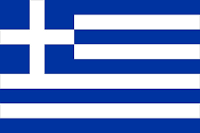


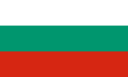
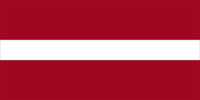
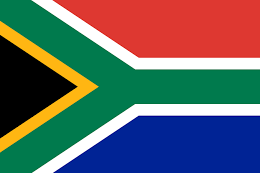
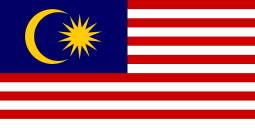



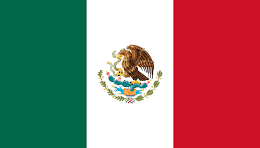
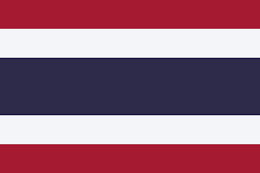
In this article by Mehul Bhat and Harmeet Singh, the authors of the book Learning Web Development with React and Bootstrap, you will learn to build two simple real-time examples:
There are many different ways to build modern web applications with JavaScript and CSS, including a lot of different tool choices, and there is a lot of new theory to learn. This article will introduce you to ReactJS and Bootstrap, which you will likely come across as you learn about modern web application development. It will then take you through the theory behind the Virtual DOM and managing the state to create interactive, reusable and stateful components ourselves and then push it to a Redux architecture. You will have to learn very carefully about the concept of props and state management and where it belongs.
If you want to learn code then you have to write code, in whichever way you feel comfortable. Try to create small components/code samples, which will give you more clarity/understanding of any technology. Now, let's see how this article is going to make your life easier when it comes to Bootstrap and ReactJS.
Facebook has really changed the way we think about frontend UI development with the introduction of React. One of the main advantages of this component-based approach is that it is easy to understand, as the view is just a function of the properties and state.
We're going to cover the following topics:
(For more resources related to this topic, see here.)
React (sometimes called React.js or ReactJS) is an open-source JavaScript library that provides a view for data rendered as HTML. Components have been used typically to render React views that contain additional components specified as custom HTML tags. React gives you a trivial virtual DOM, powerful views without templates, unidirectional data flow, and explicit mutation. It is very methodical in updating the HTML document when the data changes; and provides a clean separation of components on a modern single-page application.
From below example, we will have clear idea on normal HTML encapsulation and ReactJS custom HTML tag.
JavaScript code:
<section>
<h2>Add your Ticket</h2>
</section>
<script>
var root = document.querySelector('section').createShadowRoot();
root.innerHTML = '<style>h2{ color: red; }</style>' +
'<h2>Hello World!</h2>';
</script>
ReactJS code:
var sectionStyle = {
color: 'red'
};
var AddTicket = React.createClass({
render: function() {
return (<section><h2 style={sectionStyle}>
Hello World!</h2></section>)}
})
ReactDOM.render(<AddTicket/>, mountNode);
As your app comes into existence and develops, it's advantageous to ensure that your components are used in the right manner. The React app consists of reusable components, which makes code reuse, testing, and separation of concerns easy.
React is not only the V in MVC, it has stateful components (stateful components remembers everything within this.state). It handles mapping from input to state changes, and it renders components. In this sense, it does everything that an MVC does.
Let's look at React's component life cycle and its different levels. Observe the following screenshot:
React isn't an MVC framework; it's a library for building a composable user interface and reusable components. React used at Facebook in production and https://www.instagram.com/ is entirely built on React.
When we start to make an application with ReactJS, we need to do some setup, which just involves an HTML page and includes a few files. First, we create a directory (folder) called Chapter 1. Open it up in any code editor of your choice. Create a new file called index.html directly inside it and add the following HTML5 boilerplate code:
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<title>ReactJS Chapter 1</title>
</head>
<body>
<!--[if lt IE 8]>
<p class="browserupgrade">You are using an <strong>outdated</strong> browser.
Please <a href="http://browsehappy.com/">upgrade your browser</a> to improve your experience.</p>
<![endif]-->
<!-- Add your site or application content here -->
<p>Hello world! This is HTML5 Boilerplate.</p>
</body>
</html>
This is a standard HTML page that we can update once we have included the React and Bootstrap libraries.
Now we need to create couple of folders inside the Chapter 1 folder named images, css, and js (JavaScript) to make your application manageable. Once you have completed the folder structure it will look like this:
Once we have finished creating the folder structure, we need to install both of our frameworks, ReactJS and Bootstrap. It's as simple as including JavaScript and CSS files in your page. We can do this via a content delivery network (CDN), such as Google or Microsoft, but we are going to fetch the files manually in our application so we don't have to be dependent on the Internet while working offline.
First, we have to go to this URL https://facebook.github.io/react/ and hit the download button.
This will give you a ZIP file of the latest version of ReactJS that includes ReactJS library files and some sample code for ReactJS.
For now, we will only need two files in our application: react.min.js and react-dom.min.js from the build directory of the extracted folder.
Here are a few steps we need to follow:
<script type="text/js" src="js/react.min.js"></script>
<script type="text/js" src="js/react-dom.min.js"></script>
<script type="text/js" src="js/react.min.js"></script>
<script type="text/js" src="js/react-dom.min.js"></script>
<script type="text/js" src="js/browser.min.js"></script>
Here is what the final structure of your js folder will look like:
Bootstrap is an open source frontend framework maintained by Twitter for developing responsive websites and web applications. It includes HTML, CSS, and JavaScript code to build user interface components. It's a fast and easy way to develop a powerful mobile first user interface.
The Bootstrap grid system allows you to create responsive 12-column grids, layouts, and components. It includes predefined classes for easy layout options (fixed-width and full width). Bootstrap has a dozen pre-styled reusable components and custom jQuery plugins, such as button, alerts, dropdown, modal, tooltip tab, pagination, carousal, badges, icons, and much more.
Now, we need to install Bootstrap. Visit http://getbootstrap.com/getting-started/#download and hit on the Download Bootstrap button.
This includes the compiled and minified version of css and js for our app; we just need the CSS bootstrap.min.css and fonts folder. This style sheet will provide you with the look and feel of all of the components, and is responsive layout structure for our application. Previous versions of Bootstrap included icons as images but, in version 3, icons have been replaced as fonts. We can also customize the Bootstrap CSS style sheet as per the component used in your application:
<link rel="stylesheet" href="css/bootstrap.min.css">
That's it. Now we can open up index.html again, but this time in your browser, to see what we are working with. Here is the code that we have written so far:
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<title>ReactJS Chapter 1</title>
<link rel="stylesheet" href="css/bootstrap.min.css">
<script type="text/javascript" src="js/react.min.js"></script>
<script type="text/javascript" src="js/react-dom.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.8.23/browser.min.js"></script>
</head>
<body>
<!--[if lt IE 8]>
<p class="browserupgrade">You are using an <strong>outdated</strong> browser.
Please <a href="http://browsehappy.com/">upgrade your browser</a> to improve your experience.</p>
<![endif]-->
<!-- Add your site or application content here -->
</body>
</html>
So now we've got the ReactJS and Bootstrap style sheet and in there we've initialized our app. Now let's start to write our first Hello World app using reactDOM.render().
The first argument of the ReactDOM.render method is the component we want to render and the second is the DOM node to which it should mount (append) to:
ReactDOM.render( ReactElement element, DOMElement container, [function callback])
In order to translate it to vanilla JavaScript, we use wraps in our React code, <script type"text/babel">, tag that actually performs the transformation in the browser.
Let's start out by putting one div tag in our body tag:
<div id="hello"></div>
Now, add the script tag with the React code:
<script type="text/babel">
ReactDOM.render(
<h1>Hello, world!</h1>,
document.getElementById('hello')
);
</script>
Let's open the HTML page in your browser. If you see Hello, world! in your browser then we are on good track.
In the preceding screenshot, you can see it shows the Hello, world! in your browser. That's great. We have successfully completed our setup and built our first Hello World app. Here is the full code that we have written so far:
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<title>ReactJS Chapter 1</title>
<link rel="stylesheet" href="css/bootstrap.min.css">
<script type="text/javascript" src="js/react.min.js"></script>
<script type="text/javascript" src="js/react-dom.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-core/5.8.23/browser.min.js"></script>
</head>
<body>
<!--[if lt IE 8]>
<p class="browserupgrade">You are using an <strong>outdated</strong> browser.
Please <a href="http://browsehappy.com/">upgrade your browser</a> to improve your experience.</p>
<![endif]-->
<!-- Add your site or application content here -->
<div id="hello"></div>
<script type="text/babel">
ReactDOM.render(
<h1>Hello, world!</h1>,
document.getElementById('hello')
);
</script>
</body>
</html>
We have completed our first Hello World app with React and Bootstrap and everything looks good and as expected. Now it's time do more and create one static login form, applying the Bootstrap look and feel to it. Bootstrap is a great way to make you app responsive grid system for different mobile devices and apply the fundamental styles on HTML elements with the inclusion of a few classes and div's.
The responsive grid system is an easy, flexible, and quick way to make your web application responsive and mobile first that appropriately scales up to 12 columns per device and viewport size.
First, let's start to make an HTML structure to follow the Bootstrap grid system.
Create a div and add a className .container for (fixed width) and .container-fluid for (full width). Use the className attribute instead of using class:
<div className="container-fluid"></div>
As we know, class and for are discouraged as XML attribute names. Moreover, these are reserved words in many JavaScript libraries so, to have clear difference and identical understanding, instead of using class and for, we can use className and htmlFor create a div and adding the className row. The row must be placed within .container-fluid:
<div className="container-fluid">
<div className="row"></div>
</div>
Now create columns that must be immediate children of a row:
<div className="container-fluid">
<div className="row">
<div className="col-lg-6"></div>
</div>
</div>
.row and .col-xs-4 are predefined classes that available for quickly making grid layouts.
Add the h1 tag for the title of the page:
<div className="container-fluid">
<div className="row">
<div className="col-sm-6">
<h1>Login Form</h1>
</div>
</div>
</div>
Grid columns are created by the given specified number of col-sm-* of 12 available columns. For example, if we are using a four column layout, we need to specify to col-sm-3 lead-in equal columns.
Col-sm-* | Small devices |
Col-md-* | Medium devices |
Col-lg-* | Large devices |
We are using the col-sm-* prefix to resize our columns for small devices. Inside the columns, we need to wrap our form elements label and input tags into a div tag with the form-group class:
<div className="form-group">
<label for="emailInput">Email address</label>
<input type="email" className="form-control" id="emailInput" placeholder="Email"/>
</div>
Forget the style of Bootstrap; we need to add the form-control class in our input elements. If we need extra padding in our label tag then we can add the control-label class on the label.
Let's quickly add the rest of the elements. I am going to add a password and submit button.
In previous versions of Bootstrap, form elements were usually wrapped in an element with the form-actions class. However, in Bootstrap 3, we just need to use the same form-group instead of form-actions.
Here is our complete HTML code:
<div className="container-fluid">
<div className="row">
<div className="col-lg-6">
<form>
<h1>Login Form</h1>
<hr/>
<div className="form-group">
<label for="emailInput">Email address</label>
<input type="email" className="form-control" id="emailInput" placeholder="Email"/>
</div>
<div className="form-group">
<label for="passwordInput">Password</label>
<input type="password" className="form- control" id="passwordInput" placeholder="Password"/>
</div>
<button type="submit" className="btn btn-default col-xs-offset-9 col-xs-3">Submit</button>
</form>
</div>
</div>
</div>
Now create one object inside the var loginFormHTML script tag and assign this HTML them:
Var loginFormHTML = <div className="container-fluid">
<div className="row">
<div className="col-lg-6">
<form>
<h1>Login Form</h1>
<hr/>
<div className="form-group">
<label for="emailInput">Email address</label>
<input type="email" className="form-control" id="emailInput" placeholder="Email"/>
</div>
<div className="form-group">
<label for="passwordInput">Password</label>
<input type="password" className="form- control" id="passwordInput" placeholder="Password"/>
</div>
<button type="submit" className="btn btn-default col-xs-offset-9 col-xs-3">Submit</button>
</form>
</div>
</div>
We will pass this object in the React.DOM()method instead of directly passing the HTML:
ReactDOM.render(LoginformHTML,document.getElementById('hello'));
Our form is ready. Now let's see how it looks in the browser:
The compiler is unable to parse our HTML because we have not enclosed one of the div tags properly. You can see in our HTML that we have not closed the wrapper container-fluid at the end. Now close the wrapper tag at the end and open the file again in your browser.
Note: Whenever you hand-code (write) your HTML code, please double check your "Start Tag" and "End Tag". It should be written/closed properly, otherwise it will break your UI/frontend look and feel.
Here is the HTML after closing the div tag:
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<title>ReactJS Chapter 1</title>
<link rel="stylesheet" href="css/bootstrap.min.css">
<script type="text/javascript" src="js/react.min.js"></script>
<script type="text/javascript" src="js/react-dom.min.js"></script>
<script src="js/browser.min.js"></script>
</head>
<body>
<!-- Add your site or application content here -->
<div id="loginForm"></div>
<script type="text/babel">
var LoginformHTML =
<div className="container-fluid">
<div className="row">
<div className="col-lg-6">
<form>
<h1>Login Form</h1>
<hr/>
<div className="form-group">
<label for="emailInput">Email address</label>
<input type="email" className="form-control" id="emailInput" placeholder="Email"/>
</div>
<div className="form-group">
<label for="passwordInput">Password</label>
<input type="password" className="form-control" id="passwordInput" placeholder="Password"/>
</div>
<button type="submit" className="btn btn-default col-xs-offset-9 col-xs-3">Submit</button>
</form>
</div>
</div>
</div>
ReactDOM.render(LoginformHTML,document.getElementById('loginForm');
</script>
</body>
</html>
Now, you can check your page on browser and you will be able to see the form with below shown look and feel.
Now it's working fine and looks good. Bootstrap also provides two additional classes to make your elements smaller and larger: input-lg and input-sm. You can also check the responsive behavior by resizing the browser.
That's look great. Our small static login form application is ready with responsive behavior. Some of the benefits are:
Our simple static login form application and Hello World examples are looking great and working exactly how they should, so let's recap what we've learned in the this article.
To begin with, we saw just how easy it is to get ReactJS and Bootstrap installed with the inclusion of JavaScript files and a style sheet. We also looked at how the React application is initialized and started building our first form application.
The Hello World app and form application which we have created demonstrates some of React's and Bootstrap's basic features such as the following:
With Bootstrap, we worked towards having a responsive grid system for different mobile devices and applied the fundamental styles of HTML elements with the inclusion of a few classes and divs.
We also saw the framework's new mobile-first responsive design in action without cluttering up our markup with unnecessary classes or elements.