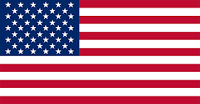

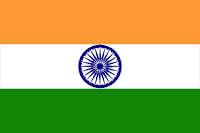
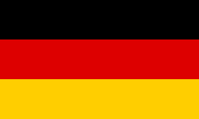
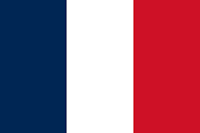

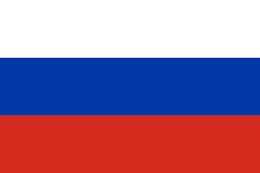
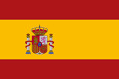



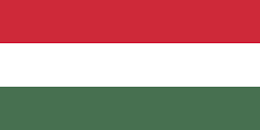

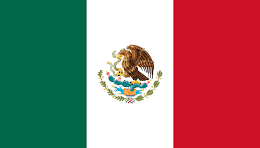
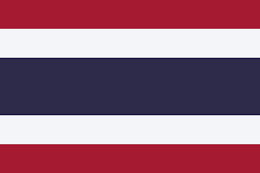
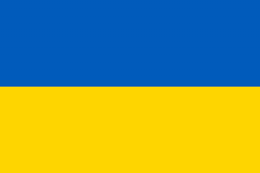
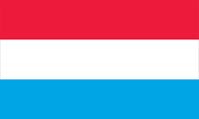

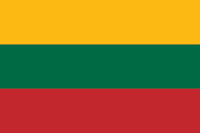

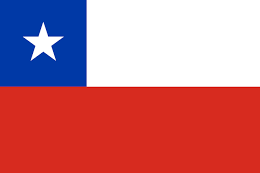

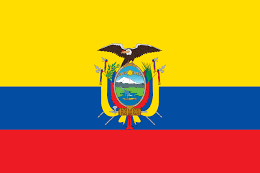

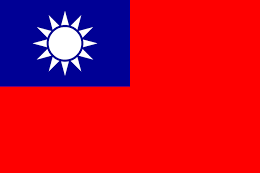

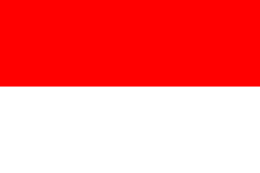
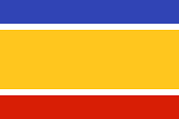
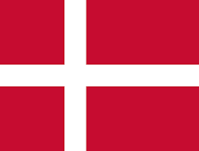
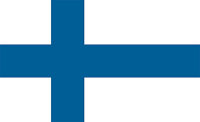


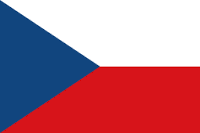
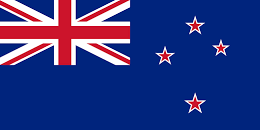
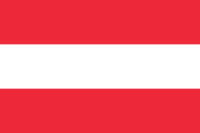
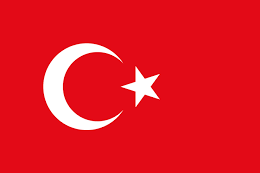
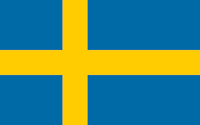
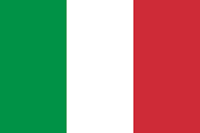
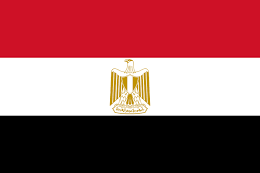

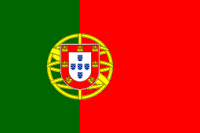
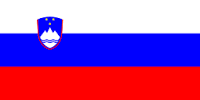

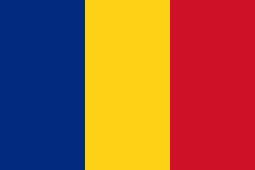
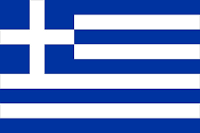

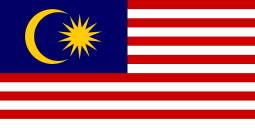
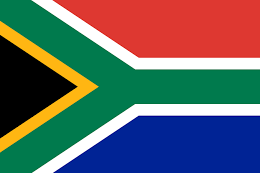

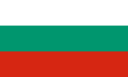
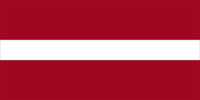


This article is an excerpt from the book, Building AI Applications with ChatGPT API, by Martin Yanev. This book will help you master ChatGPT, Whisper, and DALL-E APIs by building nine innovative AI projects
In this section, we will dive into the implementation of the key functions within the essay generator application. These functions are responsible for generating the essay based on user input and saving the generated essay to a file. By understanding the code, you will be able to grasp the inner workings of the application and gain insight into how the essay generation and saving processes are accomplished.
We will begin by exploring the generate_essay()
function. This function will retrieve the topic entered by the user from the input field. It will then set the engine type for the OpenAI API, create a prompt using the topic, and make a request to the OpenAI API for essay generation. The response received from the API will contain the generated essay, which will be extracted and displayed in the essay output area of the application. To add that functionality, simply remove the pass placeholder and follow the code below.
def generate_essay(self):
topic = self.topic_input.text()
length = 500
engine = "text-davinci-003"
prompt = f"Write an {length/1.5} words essay on the following topic: {topic} \n\n"
response = openai.Completion.create(engine=engine, prompt=prompt, max_tokens=length)
essay = response.choices[0].text
self.essay_output.setText(essay)
Here, we retrieve the topic entered by the user from the topic_input
QLineEdit widget and assign it to the topic variable using the text()
method. This captures the user's chosen topic for the essay. For now, we can define the length variable and set it to 500. This indicates the desired length of the generated essay. We will modify this value later by adding a dropdown menu with different token sizes to generate essays of different lengths.
We can also specify the engine used for the OpenAI API to be text-davinci-003
, which will generate the essay. You can adjust this value to utilize different language models or versions based on your requirements. We can also create the prompt variable, which is a string containing the prompt for the essay generation.
It is constructed by concatenating the text Write a {length/1.5
} essay on the following topic: where the length/1.5 variable specifies how many words our essay should be. We need to divide the tokens number by 1.5, as 1 word in English represents about 1.5 tokes. After specifying the instructions, we can pass the topic variable to the prompt. This prompt serves as the initial input for the essay generation process and provides context for the generated essay.
Once all variables are defined, we make a request to the ChatGPT API with the specified engine, prompt, and the maximum number of tokens (in this case, 500). The API processes the prompt and generates a response, which is stored in the response variable. From the response, we extract the generated essay by accessing the text attribute of the first choice. This represents the generated text of the essay. Finally, we can pass the AI response to the essay_output, displaying it in the user interface for the user to read and interact with.
Moving on, we will examine the save_essay()
function. This function will retrieve the topic and the generated essay. It will utilize the docx library to create a new Word document and add the final essay to the document. The document will then be saved with the filename based on the provided topic, resulting in a Word document that contains the generated essay. After removing the pass keyword, you can implement the described functionality using the code snippet below.
def save_essay(self):
topic = self.topic_input.text()
final_text = self.essay_output.toPlainText()
document = docx.Document()
document.add_paragraph(final_text)
document.save(topic + ".docx")
Here we retrieve the text entered in the topic_input widget and assign it to the topic variable using the text() method. This captures the topic entered by the user, which will be used as the filename for the saved essay. Next, we use the toPlainText()
method on the essay_output widget to retrieve the generated essay text and assign it to the final_text variable. This ensures that the user can edit the ChatGPT-generated essay before saving it. By capturing the topic and the final text, we are now equipped to proceed with the necessary steps to save the essay to a file.
We can now use the docx library to create a new Word document by calling docx.Document(), which initializes an empty document. We then add a paragraph to the document by using the add_paragraph() method and passing in the final_text variable, which contains the generated essay text. This adds the generated essay as a paragraph to the document. We can now save the document by calling document.save()
and providing a filename constructed by concatenating the topic variable, which represents the topic entered by the user. This saves the document as a Word file with the specified filename.
You can now test your Essay Generator by running the code in PyCharm and generating an essay following the steps below (see Figure 8.3):
Figure 8.3: Essay Generator creating an “Ancient Egypt” essay
Once the essay has been saved to a Word document, you can reshare it with your peers, submit it as a school assignment or use any Word styling options on it.
This section discussed the implementation of key functions in our essay generator application using the ChatGPT API. We built the generate_essay()
method that retrieved the user’s topic input and sent a request to the ChatGPT API for generating an AI essay. We also developed the save_essay()
method that saved the generated essay in a Word document.
In the next section, we will introduce additional functionality to the essay generator application. Specifically, we will allow the user to change the number of AI tokens used for generating the essay.
In this section, we will explore how to enhance the functionality of the essay generator application by allowing users to have control over the number of tokens used when communicating with ChatGPT. By enabling this feature, users will be able to generate essays of different lengths, tailored to their specific needs or preferences. Currently, our application has a fixed value of 500 tokens, but we will modify it to include a dropdown menu that provides different options for token sizes.
To implement this functionality, we will make use of a dropdown menu that presents users with a selection of token length options. By selecting a specific value from the dropdown, users can indicate their desired length for the generated essay. We will integrate this feature seamlessly into the existing application, empowering users to customize their essay-generation experience.
Let's delve into the code snippet that will enable users to control the token length. You can add that code inside the initUI()
methods, just under the essay_output resizing:
self.essay_output.resize(1100, 500)
length_label = QLabel('Select Essay Length:', self)
length_label.move(327, 40)
self.length_dropdown = QComboBox(self)
self.length_dropdown.move(320, 60)
self.length_dropdown.addItems(["500", "1000", "2000", "3000", "4000"])
The code above introduces a QLabel, length_label
, which serves as a visual indication for the purpose of the dropdown menu. It displays the text Select Essay Length to inform users about the functionality.
Next, we create a QcomboBox length_dropdown which provides users with a dropdown menu to choose the desired token length. It is positioned below the length_label
using the move() method. The addItems()
function is then used to populate the dropdown menu with a list of token length options, ranging from 500 to 4000 tokens. Users can select their preferred length from this list.
The final step is to implement the functionality that allows users to control the number of tokens used when generating the essay, we need to modify the generate_essay()
function. The modified code should be the following:
def generate_essay(self):
topic = self.topic_input.text()
length = int(self.length_dropdown.currentText())
engine = "text-davinci-003"
prompt = f"Write an {length/1.5} words essay on the following topic: {topic} \n\n"
response = openai.Completion.create(engine=engine, prompt=prompt, max_tokens=length)
essay = response.choices[0].text
self.essay_output.setText(essay)
In the modified code, the length variable is updated to retrieve the selected token length from the length_dropdown dropdown menu. The currentText()
method is used to obtain the currently selected option as a string, which is then converted to an integer using the int() function. This allows the chosen token length to be assigned to the length variable dynamically.
By making this modification, the generate_essay()
the function will utilize the user-selected token length when making the request to the ChatGPT API for essay generation. This ensures that the generated essay will have the desired length specified by the user through the dropdown menu.
We can now click on the Run button in PyCharm and verify that the Dropdown menu works properly. As shown in Figure 8.4, a click on the Dropdown menu will show users all options specified by the addItems()
function.
Figure 8.4: Controlling essay length.
The user will be able to choose a token amount between 500 and 4000. Now you can select the 4000 tokens option, resulting in a longer length of the generated essay. We can follow the steps from our previous example and verify that the ChatGPT API generates a longer essay for “Ancient Egypt” when the number of tokens is increased from 500 to 4000.
This is how you can enhance the functionality of an essay generator application by allowing users to control the number of tokens used when communicating with ChatGPT. By selecting a specific value from the dropdown menu, users can now indicate their desired length for the generated essay. We achieved that by using the QComboBox to create the dropdown menu itself. The modified generate_essay() method retrieved the selected token length from the dropdown menu and dynamically assigned it to the length variable.
In conclusion, leveraging the capabilities of ChatGPT API to enhance essay generation opens up a world of interactive creativity. By incorporating practical examples and step-by-step instructions, we have explored how to generate essay-generating elements and make them interact seamlessly with ChatGPT. This powerful combination allows for the production of compelling, coherent, and engaging essays effortlessly. With the ever-evolving potential of AI, the future of essay generation holds immense possibilities. By embracing these techniques, writers and researchers can unlock their full creative potential and revolutionize the way we generate written content.
Martin Yanev is an experienced Software Engineer who has worked in the aerospace and medical industries for over 8 years. He specializes in developing and integrating software solutions for air traffic control and chromatography systems. Martin is a well-respected instructor with over 280,000 students worldwide, and he is skilled in using frameworks like Flask, Django, Pytest, and TensorFlow. He is an expert in building, training, and fine-tuning AI systems with the full range of OpenAI APIs. Martin has dual master's degrees in Aerospace Systems and Software Engineering, which demonstrates his commitment to both practical and theoretical aspects of the industry.